700
|
How can I filter the check-boxes (method 1)
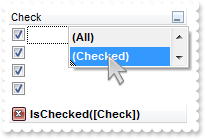
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
With .Columns.Add("Check")
With .Editor
.EditType = 19
.Option(17) = 1
End With
.DisplayFilterButton = True
.DisplayFilterPattern = False
.FilterType = 6
End With
With .Items
.AddItem True
.AddItem True
.AddItem False
.AddItem True
.AddItem False
.AddItem True
.AddItem False
End With
End With
End Function
</SCRIPT>
</BODY>
|
699
|
How can add a button to control
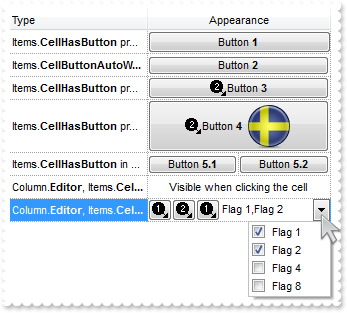
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_ButtonClick(Item,ColIndex,Key)
With Grid1
alert( "ButtonClick" )
alert( .Items.CellCaption(Item,ColIndex) )
alert( Key )
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.DefaultItemHeight = 22
.HeaderHeight = 22
.Appearance = 0
.DrawGridLines = -2
.ScrollBySingleLine = False
.Images "gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTq" & _
"lVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m" & _
"0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/y" & _
"NAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA="
.HTMLPicture("pic1") = "c:\exontrol\images\auction.gif"
With .Columns
With .Add("Type")
.Width = 48
.Def(17) = 1
End With
With .Add("Appearance")
.Def(17) = 1
.Alignment = 1
.HeaderAlignment = 1
End With
End With
With .Items
h = .AddItem("Items.<b>CellHasButton</b> property")
.CellValue(h,1) = "Button <b>1</b>"
.CellHasButton(h,1) = True
h = .AddItem("Items.<b>CellButtonAutoWidth</b> property")
.CellValue(h,1) = " Button <b>2</b> "
.CellHasButton(h,1) = True
.CellButtonAutoWidth(h,1) = True
h = .AddItem("Items.<b>CellHasButton</b> property")
.CellValue(h,1) = " <img>2</img>Button <b>3</b> "
.CellHasButton(h,1) = True
.CellButtonAutoWidth(h,1) = True
h = .AddItem("Items.<b>CellHasButton</b> property")
.ItemHeight(h) = 32
.CellValue(h,1) = " <img>2</img>Button <b>4</b> <img>pic1</img> "
.CellHasButton(h,1) = True
.CellButtonAutoWidth(h,1) = True
h = .AddItem("Items.<b>CellHasButton</b> in splitted cells")
.CellValue(h,1) = " Button <b>5.1</b> "
.CellHasButton(h,1) = True
.CellButtonAutoWidth(h,1) = True
s = .SplitCell(h,1)
.CellValue(0,s) = " Button <b>5.2</b> "
.CellHasButton(0,s) = True
.CellButtonAutoWidth(0,s) = True
h = .AddItem("Column.<b>Editor</b>, Items.<b>CellEditor</b>")
.CellValue(h,1) = "Visible when clicking the cell"
With .CellEditor(h,1)
.EditType = 1
.AddButton "B1",1,0,"This is a bit of text that's shown when the cursor hovers the button B1"
.AddButton "B3",2,1,"This is a bit of text that's shown when the cursor hovers the button B3"
.AddButton "B4",1,1,"This is a bit of text that's shown when the cursor hovers the button B4"
.ButtonWidth = 24
End With
h = .AddItem("Column.<b>Editor</b>, Items.<b>CellEditor</b>")
.CellValue(h,1) = 3
With .CellEditor(h,1)
.EditType = 6
.AddItem 1,"Flag 1"
.AddItem 2,"Flag 2"
.AddItem 4,"Flag 4"
.AddItem 8,"Flag 8"
.AddButton "C1",1,0,"This is a bit of text that's shown when the cursor hovers the button C1"
.AddButton "C3",2,0,"This is a bit of text that's shown when the cursor hovers the button C2"
.AddButton "C4",1,0,"This is a bit of text that's shown when the cursor hovers the button C3"
.ButtonWidth = 24
End With
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
698
|
The item is not getting selected when clicking the cell's checkbox. What should I do
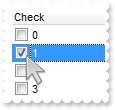
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_CellStateChanged(Item,ColIndex)
With Grid1
.Items.SelectItem(Item) = True
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.Columns.Add("Check").Def(0) = True
With .Items
.AddItem 0
.AddItem 1
.AddItem 2
.AddItem 3
End With
End With
End Function
</SCRIPT>
</BODY>
|
697
|
Is it possible to limit the height of the item while resizing
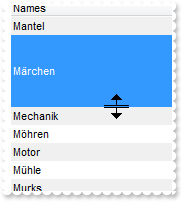
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_AddItem(Item)
With Grid1
.Items.ItemMinHeight(Item) = 18
.Items.ItemMaxHeight(Item) = 72
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ItemsAllowSizing = -1
.ScrollBySingleLine = False
.BackColorAlternate = RGB(240,240,240)
.Columns.Add "Names"
With .Items
.AddItem "Mantel"
.AddItem "Mechanik"
.AddItem "Motor"
.AddItem "Murks"
.AddItem "Märchen"
.AddItem "Möhren"
.AddItem "Mühle"
End With
.Columns.Item(0).SortOrder = 1
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
696
|
Is it possible to copy the hierarchy of the control using the GetItems method
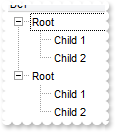
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.LinesAtRoot = -1
.Columns.Add "Def"
With .Items
h = .AddItem("Root")
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
End With
.PutItems .GetItems(-1)
End With
End Function
</SCRIPT>
</BODY>
|
695
|
Is it possible to auto-numbering the children items but still keeps the position after filtering
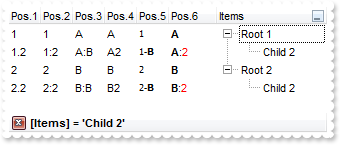
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
With .Columns.Add("Items")
.DisplayFilterButton = True
.FilterType = 240
.Filter = "Child 2"
End With
With .Columns.Add("Pos.1")
.FormatColumn = "1 ropos ''"
.Position = 0
.Width = 32
.AllowSizing = False
End With
With .Columns.Add("Pos.2")
.FormatColumn = "1 ropos ':'"
.Position = 1
.Width = 32
.AllowSizing = False
End With
With .Columns.Add("Pos.3")
.FormatColumn = "1 ropos ':|A-Z'"
.Position = 2
.Width = 32
.AllowSizing = False
End With
With .Columns.Add("Pos.4")
.FormatColumn = "1 ropos '|A-Z|'"
.Position = 3
.Width = 32
.AllowSizing = False
End With
With .Columns.Add("Pos.5")
.FormatColumn = "'<font Tahoma;7>' + 1 ropos '-<b>||A-Z'"
.Def(17) = 1
.Position = 4
.Width = 32
.AllowSizing = False
End With
With .Columns.Add("Pos.6")
.FormatColumn = "'<b>'+ 1 ropos '</b>:<fgcolor=FF0000>|A-Z|'"
.Def(17) = 1
.Position = 5
.Width = 48
.AllowSizing = False
End With
With .Items
h = .AddItem("Root 1")
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
.ExpandItem(h) = True
h = .AddItem("Root 2")
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
End With
.ApplyFilter
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
694
|
Is it possible to auto-numbering the children items too
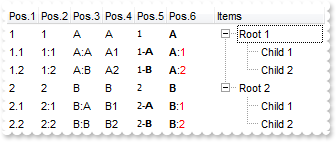
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
.Columns.Add "Items"
With .Columns.Add("Pos.1")
.FormatColumn = "1 rpos ''"
.Position = 0
.Width = 32
.AllowSizing = False
End With
With .Columns.Add("Pos.2")
.FormatColumn = "1 rpos ':'"
.Position = 1
.Width = 32
.AllowSizing = False
End With
With .Columns.Add("Pos.3")
.FormatColumn = "1 rpos ':|A-Z'"
.Position = 2
.Width = 32
.AllowSizing = False
End With
With .Columns.Add("Pos.4")
.FormatColumn = "1 rpos '|A-Z|'"
.Position = 3
.Width = 32
.AllowSizing = False
End With
With .Columns.Add("Pos.5")
.FormatColumn = "'<font Tahoma;7>' + 1 rpos '-<b>||A-Z'"
.Def(17) = 1
.Position = 4
.Width = 32
.AllowSizing = False
End With
With .Columns.Add("Pos.6")
.FormatColumn = "'<b>'+ 1 rpos '</b>:<fgcolor=FF0000>|A-Z|'"
.Def(17) = 1
.Position = 5
.Width = 48
.AllowSizing = False
End With
With .Items
h = .AddItem("Root 1")
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
.ExpandItem(h) = True
h = .AddItem("Root 2")
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
693
|
Is it possible to cancel or discard the values during validation
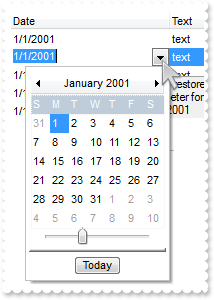
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_ValidateValue(Item,ColIndex,NewValue,Cancel)
With Grid1
alert( "ValidateValue" )
alert( NewValue )
alert( "Change the Cancel parameter for ValidateValue event to accept/decline the newly value. " )
alert( "The DiscardValidateValue restores back the previously values." )
.DiscardValidateValue
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.CauseValidateValue = -1
.Columns.Add("Date").Editor.EditType = 7
.Columns.Add("Text").Editor.EditType = 1
With .Items
.CellValue(.AddItem(#1/1/2001#),1) = "text"
.CellValue(.AddItem(#1/1/2001#),1) = "text"
.CellValue(.AddItem(#1/1/2001#),1) = "text"
.CellValue(.AddItem(#1/1/2001#),1) = "text"
.CellValue(.AddItem(#1/1/2001#),1) = "text"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
692
|
Is it possible to validate the values of the cells only when user leaves the focused item
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_ValidateValue(Item,ColIndex,NewValue,Cancel)
With Grid1
alert( "ValidateValue" )
alert( NewValue )
alert( "Change the Cancel parameter for ValidateValue event to accept/decline the newly value. " )
Cancel = True
alert( "You can not leave the item/record until the Cancel is False." )
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.CauseValidateValue = 1
.Columns.Add("Date").Editor.EditType = 7
.Columns.Add("Text").Editor.EditType = 1
With .Items
.CellValue(.AddItem(#1/1/2001#),1) = "text"
.CellValue(.AddItem(#1/1/2001#),1) = "text"
.CellValue(.AddItem(#1/1/2001#),1) = "text"
.CellValue(.AddItem(#1/1/2001#),1) = "text"
.CellValue(.AddItem(#1/1/2001#),1) = "text"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
691
|
We would like to validate the values of the cells. Is it possible
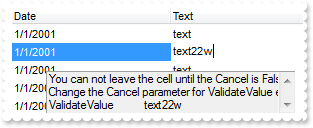
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_ValidateValue(Item,ColIndex,NewValue,Cancel)
With Grid1
alert( "ValidateValue" )
alert( NewValue )
alert( "Change the Cancel parameter for ValidateValue event to accept/decline the newly value." )
Cancel = True
alert( "You can not leave the cell until the Cancel is False." )
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.CauseValidateValue = -1
.Columns.Add("Date").Editor.EditType = 7
.Columns.Add("Text").Editor.EditType = 1
With .Items
.CellValue(.AddItem(#1/1/2001#),1) = "text"
.CellValue(.AddItem(#1/1/2001#),1) = "text"
.CellValue(.AddItem(#1/1/2001#),1) = "text"
.CellValue(.AddItem(#1/1/2001#),1) = "text"
.CellValue(.AddItem(#1/1/2001#),1) = "text"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
690
|
Is there any way to add auto-numbering

<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
With .Columns
.Add "Items"
With .Add("Pos")
.FormatColumn = "1 pos ''"
.Position = 0
End With
End With
With .Items
.AddItem "Item 1"
.AddItem "Item 2"
.AddItem "Item 3"
End With
End With
End Function
</SCRIPT>
</BODY>
|
689
|
Does your control supports multiple lines tooltip
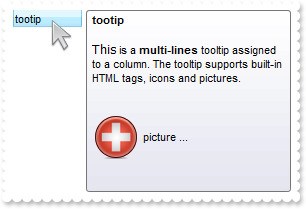
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.HTMLPicture("pic1") = "c:\exontrol\images\zipdisk.gif"
.ToolTipDelay = 1
.Columns.Add("tootip").ToolTip = "<br><font Tahoma;10>This</font> is a <b>multi-lines</b> tooltip assigned to a column. The tooltip supports built-in HTML tags, " & _
"icons and pictures.<br><br><br><img>pic1</img> picture ... <br><br>"
End With
End Function
</SCRIPT>
</BODY>
|
688
|
How can I prevent highlighting the column from the cursor - point

<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.VisualAppearance.Add 1,"gBFLBCJwBAEHhEJAEGg4BI0IQAAYAQGKIYBkAKBQAGaAoDDUOQzQwAAxDKKUEwsACEIrjKCYVgOHYYRrIMYgBCMJhLEoaZLhEZRQiqDYtRDFQBSDDcPw/EaRZohGaYJ" & _
"gEgI="
.Background(32) = &H1000000
.Columns.Add("S").Width = 32
.Columns.Add("Level 1").LevelKey = 1
.Columns.Add("Level 2").LevelKey = 1
.Columns.Add("Level 3").LevelKey = 1
.Columns.Add("E1").Width = 32
.Columns.Add("E2").Width = 32
.Columns.Add("E3").Width = 32
.Columns.Add("E4").Width = 32
End With
End Function
</SCRIPT>
</BODY>
|
687
|
Is it possible display numbers in the same format no matter of regional settings in the control panel
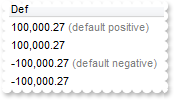
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Def").Def(17) = 1
With .Items
h = .AddItem(100000.27)
.FormatCell(h,0) = "(value format '') + ' <fgcolor=808080>(default positive)'"
h = .AddItem(100000.27)
.FormatCell(h,0) = "(value format '2|.|3|,|1|1')"
h = .AddItem(-100000.27)
.FormatCell(h,0) = "(value format '') + ' <fgcolor=808080>(default negative)'"
h = .AddItem(-100000.27)
.FormatCell(h,0) = "(value format '2|.|3|,|1|1')"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
686
|
Is it possible to add a 0 for numbers less than 1 instead .7 to show 0.8
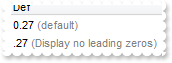
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Def").Def(17) = 1
With .Items
h = .AddItem(0.27)
.FormatCell(h,0) = "(value format '') + ' <fgcolor=808080>(default)'"
h = .AddItem(0.27)
.FormatCell(h,0) = "(value format '|||||0') + ' <fgcolor=808080>(Display no leading zeros)'"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
685
|
How can I specify the format for negative numbers
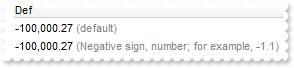
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Def").Def(17) = 1
With .Items
h = .AddItem(-100000.27)
.FormatCell(h,0) = "(value format '') + ' <fgcolor=808080>(default)'"
h = .AddItem(-100000.27)
.FormatCell(h,0) = "(value format '||||1') + ' <fgcolor=808080>(Negative sign, number; for example, -1.1)'"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
684
|
Is it possible to change the grouping character when display numbers
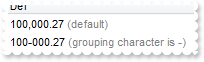
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Def").Def(17) = 1
With .Items
h = .AddItem(100000.27)
.FormatCell(h,0) = "(value format '') + ' <fgcolor=808080>(default)'"
h = .AddItem(100000.27)
.FormatCell(h,0) = "(value format '|||-') + ' <fgcolor=808080>(grouping character is -)'"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
683
|
How can I display numbers with 2 digits in each group
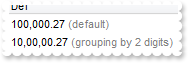
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Def").Def(17) = 1
With .Items
h = .AddItem(100000.27)
.FormatCell(h,0) = "(value format '') + ' <fgcolor=808080>(default)'"
h = .AddItem(100000.27)
.FormatCell(h,0) = "(value format '||2') + ' <fgcolor=808080>(grouping by 2 digits)'"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
682
|
How can I display my numbers using a different decimal separator
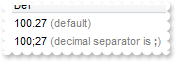
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Def").Def(17) = 1
With .Items
h = .AddItem(100.27)
.FormatCell(h,0) = "(value format '') + ' <fgcolor=808080>(default)'"
h = .AddItem(100.27)
.FormatCell(h,0) = "(value format '|;') + ' <fgcolor=808080>(decimal separator is <b>;</b>)'"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
681
|
Is it possible to display the numbers using 3 (three) digits
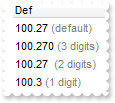
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Def").Def(17) = 1
With .Items
h = .AddItem(100.27)
.FormatCell(h,0) = "(value format '') + ' <fgcolor=808080>(default)'"
h = .AddItem(100.27)
.FormatCell(h,0) = "(value format '3') + ' <fgcolor=808080>(3 digits)'"
h = .AddItem(100.27)
.FormatCell(h,0) = "(value format 2) + ' <fgcolor=808080>(2 digits)'"
h = .AddItem(100.27)
.FormatCell(h,0) = "(value format 1) + ' <fgcolor=808080>(1 digit)'"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
680
|
Is there any option to show the tooltip programmatically
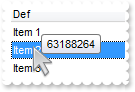
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_MouseMove(Button,Shift,X,Y)
With Grid1
.ShowToolTip .ItemFromPoint(-1,-1,c,hit),"","8","8"
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add "Def"
With .Items
.AddItem "Item 1"
.AddItem "Item 2"
.AddItem "Item 3"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
679
|
How can I specify the column's width to be the same for all columns

<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
With .Columns
.Add "A"
.Add "B"
.Add "C"
End With
.DrawGridLines = -1
.ColumnAutoResize = True
End With
End Function
</SCRIPT>
</BODY>
|
678
|
How can I set the column's width to my desired width

<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.ColumnAutoResize = False
With .Columns
.Add("A").Width = 128
.Add("B").Width = 128
End With
.DrawGridLines = -1
End With
End Function
</SCRIPT>
</BODY>
|
677
|
Is it possible to format numbers
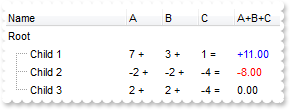
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.MarkSearchColumn = False
With .Columns
.Add "Name"
With .Add("A")
.SortType = 1
.AllowSizing = False
.Width = 36
.FormatColumn = "len(value) ? value + ' +'"
.Editor.EditType = 4
End With
With .Add("B")
.SortType = 1
.AllowSizing = False
.Width = 36
.FormatColumn = "len(value) ? value + ' +'"
.Editor.EditType = 4
End With
With .Add("C")
.SortType = 1
.AllowSizing = False
.Width = 36
.FormatColumn = "len(value) ? value + ' ='"
.Editor.EditType = 4
End With
With .Add("A+B+C")
.SortType = 1
.Width = 64
.ComputedField = "dbl(%1)+dbl(%2)+dbl(%3)"
.FormatColumn = "type(value) in (0,1) ? 'null' : ( dbl(value)<0 ? '<fgcolor=FF0000>'+ (value format '2|.|3|,|1' ) : (dbl(value)>0 ? '<fgcolor=00" & _
"00FF>+'+(value format '2|.|3|,' ): '0.00') )"
.Def(17) = 1
End With
End With
With .Items
h = .AddItem("Root")
.CellValueFormat(h,4) = 2
h1 = .InsertItem(h,,"Child 1")
.CellValue(h1,1) = 7
.CellValue(h1,2) = 3
.CellValue(h1,3) = 1
h1 = .InsertItem(h,,"Child 2")
.CellValue(h1,1) = -2
.CellValue(h1,2) = -2
.CellValue(h1,3) = -4
h1 = .InsertItem(h,,"Child 3")
.CellValue(h1,1) = 2
.CellValue(h1,2) = 2
.CellValue(h1,3) = -4
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
676
|
How can I collapse all items

<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
.Columns.Add "Items"
With .Items
h = .AddItem("Root 1")
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
h = .AddItem("Root 2")
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
.ExpandItem(0) = False
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
675
|
How can I expand all items
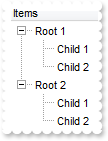
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
.Columns.Add "Items"
With .Items
h = .AddItem("Root 1")
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
h = .AddItem("Root 2")
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
.ExpandItem(0) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
674
|
Can I display a total field without having to add a child item
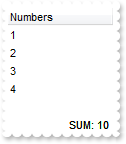
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
With .Columns.Add("Numbers")
.SortType = 1
With .Editor
.EditType = 4
.Numeric = 1
End With
End With
With .Items
.AddItem 1
.AddItem 2
.AddItem 3
.AddItem 4
.LockedItemCount(2) = 1
h = .LockedItem(2,0)
.CellValue(h,0) = "sum(all,dir,dbl(%0))"
.SortableItem(h) = False
.CellValueFormat(h,0) = 4
.CellHAlignment(h,0) = 2
.FormatCell(h,0) = "'SUM: '+value"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
673
|
Can I display the number of child items
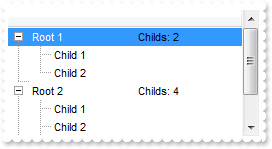
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = 1
.Columns.Add ""
With .Items
h = .AddItem("Root 1")
hx = .SplitCell(h,0)
.CellValue(0,hx) = "count(current,dir,1)"
.CellValueFormat(0,hx) = 4
.FormatCell(0,hx) = "'Childs: ' + value"
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
.ExpandItem(h) = True
h = .AddItem("Root 2")
hx = .SplitCell(h,0)
.CellValue(0,hx) = "count(current,dir,1)"
.CellValueFormat(0,hx) = 4
.FormatCell(0,hx) = "'Childs: ' + value"
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
.InsertItem h,,"Child 3"
.InsertItem h,,"Child 4"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
672
|
My field does not display the correctly computed value if I enter data using the control's editors ( concatenation of strings ). What am I doing wrong
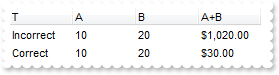
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add "T"
With .Columns.Add("A").Editor
.Numeric = True
.EditType = 4
End With
With .Columns.Add("B").Editor
.Numeric = True
.EditType = 4
End With
.Columns.Add "A+B"
With .Items
h = .AddItem("Incorrect")
.CellToolTip(h,0) = "Just type a number in the column A or B. The result will be concaternated"
.CellValue(h,1) = "10"
.CellValue(h,2) = "20"
.CellValue(h,3) = "currency(%1+%2)"
.CellValueFormat(h,3) = 2
h = .AddItem("Correct")
.CellValue(h,1) = 10
.CellValue(h,2) = 20
.CellValue(h,3) = "currency(dbl(%1)+dbl(%2))"
.CellValueFormat(h,3) = 2
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
671
|
The CellValue/CellCaption property gets the result of a computed/total field with text formatting. Is it possible to get that value without text formatting
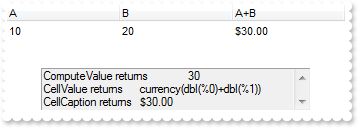
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("A").Editor.EditType = 4
.Columns.Add("B").Editor.EditType = 4
.Columns.Add "A+B"
With .Items
h = .AddItem(10)
.CellValue(h,1) = 20
.CellValueFormat(h,2) = 2
.CellValue(h,2) = "currency(dbl(%0)+dbl(%1))"
alert( "CellCaption returns " )
alert( .CellCaption(h,2) )
alert( "CellValue returns " )
alert( .CellValue(h,2) )
alert( "ComputeValue returns " )
alert( .ComputeValue("dbl(%0)+dbl(%1)",h,0,.CellValueFormat(h,2)) )
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
670
|
Can I get the result of a specified formula as your control does using the ComputedField property
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add "A"
.Columns.Add "B"
With .Items
h = .AddItem(10)
.CellValue(h,1) = 20
alert( "A+B is " )
alert( .ComputeValue("dbl(%0)+dbl(%1)",h,0,2) )
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
669
|
Is it possible to get the text without HTML formatting
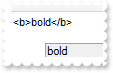
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add ""
With .Items
h = .AddItem("<b>bold</b>")
alert( .ComputeValue(.CellValue(h,0),h,0,1) )
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
668
|
Can I specify an item to be a separator
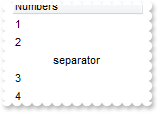
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.TreeColumnIndex = -1
.SortOnClick = 0
.Columns.Add "Numbers"
With .Items
.AddItem 1
.AddItem 2
h = .AddItem("separator")
.SelectableItem(h) = False
.ItemDivider(h) = 0
.ItemDividerLineAlignment(h) = 1
.ItemDividerLine(h) = 5
.CellHAlignment(h,0) = 1
.AddItem 3
.AddItem 4
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
667
|
How can I count only non-zero values
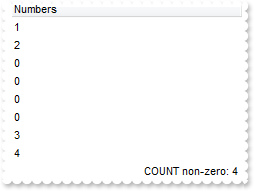
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Numbers").SortType = 1
With .Items
.AddItem 1
.AddItem 2
.AddItem 0
.AddItem 0
.AddItem 0
.AddItem 0
.AddItem 3
.AddItem 4
h = .AddItem("sum(all,dir,dbl(%0)?1:0)")
.SortableItem(h) = False
.CellValueFormat(h,0) = 4
.CellHAlignment(h,0) = 2
.FormatCell(h,0) = "'COUNT non-zero: '+value"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
666
|
How can I add a AVG ( average ) field
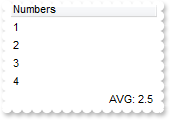
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Numbers").SortType = 1
With .Items
.AddItem 1
.AddItem 2
.AddItem 3
.AddItem 4
h = .AddItem("avg(all,dir,dbl(%0))")
.SortableItem(h) = False
.CellValueFormat(h,0) = 4
.CellHAlignment(h,0) = 2
.FormatCell(h,0) = "'AVG: '+value"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
665
|
How can I add a COUNT field
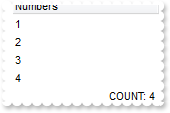
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Numbers").SortType = 1
With .Items
.AddItem 1
.AddItem 2
.AddItem 3
.AddItem 4
h = .AddItem("count(all,dir,0)")
.SortableItem(h) = False
.CellValueFormat(h,0) = 4
.CellHAlignment(h,0) = 2
.FormatCell(h,0) = "'COUNT: '+value"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
664
|
How can I add a MAX field
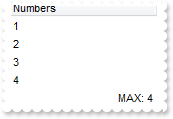
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Numbers").SortType = 1
With .Items
.AddItem 1
.AddItem 2
.AddItem 3
.AddItem 4
h = .AddItem("max(all,dir,dbl(%0))")
.SortableItem(h) = False
.CellValueFormat(h,0) = 4
.CellHAlignment(h,0) = 2
.FormatCell(h,0) = "'MAX: '+value"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
663
|
How can I add a MIN or MAX field (for numbers)
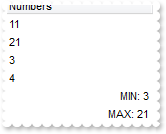
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Numbers").SortType = 1
With .Items
.AddItem 11
.AddItem 21
.AddItem 3
.AddItem 4
h = .AddItem("min(all,dir,dbl(%0))")
.SortableItem(h) = False
.CellValueFormat(h,0) = 4
.CellHAlignment(h,0) = 2
.FormatCell(h,0) = "'MIN: '+value"
h = .AddItem("max(all,dir,dbl(%0))")
.SortableItem(h) = False
.CellValueFormat(h,0) = 4
.CellHAlignment(h,0) = 2
.FormatCell(h,0) = "'MAX: '+value"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
662
|
How can I add a SUM field
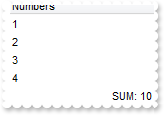
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Numbers").SortType = 1
With .Items
.AddItem 1
.AddItem 2
.AddItem 3
.AddItem 4
h = .AddItem("sum(all,dir,dbl(%0))")
.SortableItem(h) = False
.CellValueFormat(h,0) = 4
.CellHAlignment(h,0) = 2
.FormatCell(h,0) = "'SUM: '+value"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
661
|
How can I add total and subtotals fields
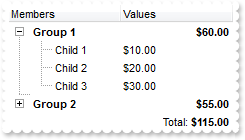
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_Change(Item,ColIndex,NewValue)
With Grid1
.Refresh
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = 1
.Columns.Add "Members"
With .Columns.Add("Values")
.FormatColumn = "currency(value)"
With .Editor
.EditType = 4
.Numeric = True
End With
End With
With .Items
h = .AddItem("Group 1")
.ItemBold(h) = True
.CellEditorVisible(h,1) = False
.CellValue(h,1) = "sum(current,dir,dbl(%1))"
.CellValueFormat(h,1) = 5 ' ValueFormatEnum.exTotalField Or ValueFormatEnum.exHTML
.CellHAlignment(h,1) = 2
.CellValue(.InsertItem(h,,"Child 1"),1) = 10
.CellValue(.InsertItem(h,,"Child 2"),1) = 20
.CellValue(.InsertItem(h,,"Child 3"),1) = 30
.ExpandItem(h) = True
h = .AddItem("Group 2")
.ItemBold(h) = True
.CellEditorVisible(h,1) = False
.CellValue(h,1) = "sum(current,dir,dbl(%1))"
.CellValueFormat(h,1) = 5 ' ValueFormatEnum.exTotalField Or ValueFormatEnum.exHTML
.CellHAlignment(h,1) = 2
.CellValue(.InsertItem(h,,"Child 1"),1) = 5
.CellValue(.InsertItem(h,,"Child 2"),1) = 15
.CellValue(.InsertItem(h,,"Child 3"),1) = 35
h = .AddItem("total")
.CellValue(h,1) = "sum(all,rec,dbl(%1))"
.CellValueFormat(h,1) = 5 ' ValueFormatEnum.exTotalField Or ValueFormatEnum.exHTML
.CellEditorVisible(h,1) = False
.FormatCell(h,1) = "'Total: <b>' + currency(value)"
.CellHAlignment(h,1) = 2
.ItemDivider(h) = 1
.ItemDividerLineAlignment(h) = 1
.ItemDividerLine(h) = 2
.SortableItem(h) = False
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
660
|
Is is possible to have subtotal items, and a grand total item
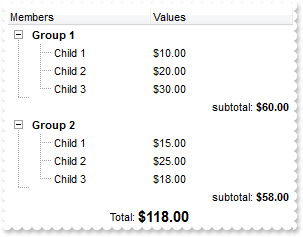
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_Change(Item,ColIndex,NewValue)
With Grid1
.Refresh
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.BackColor = RGB(255,255,255)
.LinesAtRoot = 1
.ShowFocusRect = False
.Columns.Add "Members"
With .Columns.Add("Values")
.FormatColumn = "currency(value)"
With .Editor
.EditType = 4
.Numeric = True
End With
End With
With .Items
h = .AddItem("Group 1")
.ItemBold(h) = True
.SortableItem(h) = False
.CellEditorVisible(h,1) = False
.FormatCell(h,1) = " "
.CellValue(.InsertItem(h,,"Child 1"),1) = 10
.CellValue(.InsertItem(h,,"Child 2"),1) = 20
.CellValue(.InsertItem(h,,"Child 3"),1) = 30
hT = .InsertItem(h,,"subtotal")
.CellHAlignment(hT,1) = 2
.CellEditorVisible(hT,1) = False
.CellValue(hT,1) = "sum(parent,dir,dbl(%1))"
.CellValueFormat(hT,1) = 5 ' ValueFormatEnum.exTotalField Or ValueFormatEnum.exHTML
.FormatCell(hT,1) = "'subtotal: <b>' + currency(value)"
.ItemDivider(hT) = 1
.ItemDividerLineAlignment(hT) = 1
.SortableItem(hT) = False
.SelectableItem(hT) = False
.ItemDividerLine(hT) = 3
.ExpandItem(h) = True
h = .AddItem("Group 2")
.ItemBold(h) = True
.SortableItem(h) = False
.FormatCell(h,1) = " "
.CellEditorVisible(h,1) = False
.CellValue(.InsertItem(h,,"Child 1"),1) = 15
.CellValue(.InsertItem(h,,"Child 2"),1) = 25
.CellValue(.InsertItem(h,,"Child 3"),1) = 18
hT = .InsertItem(h,,"subtotal")
.CellHAlignment(hT,1) = 2
.CellEditorVisible(hT,1) = False
.CellValue(hT,1) = "sum(parent,dir,dbl(%1))"
.CellValueFormat(hT,1) = 5 ' ValueFormatEnum.exTotalField Or ValueFormatEnum.exHTML
.FormatCell(hT,1) = "'subtotal: <b>' + currency(value)"
.ItemDivider(hT) = 1
.ItemDividerLineAlignment(hT) = 1
.ItemDividerLine(hT) = 3
.SortableItem(hT) = False
.SelectableItem(hT) = False
.ExpandItem(h) = True
h = .AddItem("total")
.CellValue(h,1) = "sum(all,rec,dbl(%1))"
.CellValueFormat(h,1) = 5 ' ValueFormatEnum.exTotalField Or ValueFormatEnum.exHTML
.CellEditorVisible(h,1) = False
.FormatCell(h,1) = "'Total: <b><font ;11>' + currency(value)"
.CellHAlignment(h,1) = 1
.ItemDivider(h) = 1
.ItemDividerLineAlignment(h) = 1
.ItemDividerLine(h) = 2
.SortableItem(h) = False
.SelectableItem(h) = False
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
659
|
Is it possible to have a total field for each column
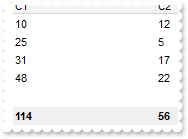
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_Change(Item,ColIndex,NewValue)
With Grid1
.Refresh
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
With .Columns.Add("C1")
With .Editor
.Numeric = -1
.EditType = 4
End With
.SortType = 1
End With
With .Columns.Add("C2")
With .Editor
.Numeric = -1
.EditType = 4
End With
.SortType = 1
End With
With .Items
.LockedItemCount(2) = 1
h = .LockedItem(2,0)
.ItemBackColor(h) = RGB(240,240,240)
.ItemBold(h) = True
.CellValue(h,0) = "sum(all,dir,dbl(%0))"
.CellValueFormat(h,0) = 4
.CellValue(h,1) = "sum(all,dir,dbl(%1))"
.CellValueFormat(h,1) = 4
End With
With .Items
.CellValue(.AddItem(10),1) = 12
.CellValue(.AddItem(25),1) = 5
.CellValue(.AddItem(31),1) = 17
.CellValue(.AddItem(48),1) = 22
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
658
|
How can I add a total field for a DataSource being used
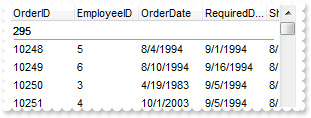
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_Change(Item,ColIndex,NewValue)
With Grid1
.Refresh
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.ColumnAutoResize = False
.ContinueColumnScroll = False
Set rs = CreateObject("ADOR.Recordset")
With rs
.Open "Orders","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\Program Files\Exontrol\ExGrid\Sample\Access\misc.accdb",3,3
End With
.DataSource = rs
With .Items
.LockedItemCount(0) = 1
h = .LockedItem(0,0)
.ItemDivider(h) = 0
.CellValueFormat(h,0) = 5 ' ValueFormatEnum.exTotalField Or ValueFormatEnum.exHTML
.CellValue(h,0) = "sum(all,dir,%1)"
End With
End With
End Function
</SCRIPT>
</BODY>
|
657
|
How can I add a total field
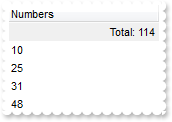
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_Change(Item,ColIndex,NewValue)
With Grid1
.Refresh
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
With .Columns.Add("Numbers")
With .Editor
.Numeric = -1
.EditType = 4
End With
.SortType = 1
End With
With .Items
.LockedItemCount(0) = 1
h = .LockedItem(0,0)
.ItemBackColor(h) = RGB(240,240,240)
.CellValue(h,0) = "sum(all,dir,dbl(%0))"
.CellValueFormat(h,0) = 4
.CellHAlignment(h,0) = 2
.FormatCell(h,0) = "'Total: '+value"
End With
With .Items
.AddItem 10
.AddItem 25
.AddItem 31
.AddItem 48
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
656
|
How can I add a total field
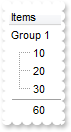
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_Change(Item,ColIndex,NewValue)
With Grid1
.Refresh
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
With .Columns.Add("Items").Editor
.EditType = 4
.Numeric = True
End With
With .Items
h = .AddItem("Group 1")
.CellEditorVisible(h,0) = False
.InsertItem h,,10
.InsertItem h,,20
.InsertItem h,,30
hT = .InsertItem(h,,"sum(parent,dir,dbl(%0))")
.CellEditorVisible(hT,0) = False
.CellValueFormat(hT,0) = 5 ' ValueFormatEnum.exTotalField Or ValueFormatEnum.exHTML
.ItemDivider(hT) = 0
.ItemDividerLineAlignment(hT) = 2
.SelectableItem(hT) = False
.SortableItem(hT) = False
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
655
|
Is it possible to specify the cell's value but still want to display some formatted text instead the value
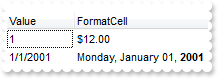
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add "Value"
.Columns.Add "FormatCell"
With .Items
h = .AddItem(1)
.CellValue(h,1) = 12
.FormatCell(h,1) = "currency(value)"
h = .AddItem(#1/1/2001#)
.CellValue(h,1) = #1/1/2001#
.CellValueFormat(h,1) = 1
.FormatCell(h,1) = "longdate(value) replace '2001' with '<b>2001</b>'"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
654
|
How can I simulate displaying groups
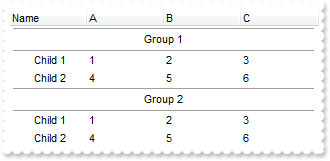
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.HasLines = 0
.ScrollBySingleLine = True
With .Columns
.Add "Name"
.Add "A"
.Add "B"
.Add "C"
End With
With .Items
h = .AddItem("Group 1")
.CellHAlignment(h,0) = 1
.ItemDivider(h) = 0
.ItemDividerLineAlignment(h) = 3
.ItemHeight(h) = 24
.SortableItem(h) = False
h1 = .InsertItem(h,,"Child 1")
.CellValue(h1,1) = 1
.CellValue(h1,2) = 2
.CellValue(h1,3) = 3
h1 = .InsertItem(h,,"Child 2")
.CellValue(h1,1) = 4
.CellValue(h1,2) = 5
.CellValue(h1,3) = 6
.ExpandItem(h) = True
h = .AddItem("Group 2")
.CellHAlignment(h,0) = 1
.ItemDivider(h) = 0
.ItemDividerLineAlignment(h) = 3
.ItemHeight(h) = 24
.SortableItem(h) = False
h1 = .InsertItem(h,,"Child 1")
.CellValue(h1,1) = 1
.CellValue(h1,2) = 2
.CellValue(h1,3) = 3
h1 = .InsertItem(h,,"Child 2")
.CellValue(h1,1) = 4
.CellValue(h1,2) = 5
.CellValue(h1,3) = 6
.ExpandItem(h) = True
End With
End With
End Function
</SCRIPT>
</BODY>
|
653
|
Is it possible to specify the cell's value but still want to display some formatted text instead the value
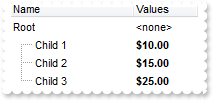
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.MarkSearchColumn = False
With .Columns
.Add "Name"
With .Add("Values")
.SortType = 1
.AllowSizing = False
.Width = 64
.FormatColumn = "((0:=dbl(value)) < 10? '<fgcolor=808080><font ;7>' :'<b>') + currency(=:0)"
.Def(17) = 1
End With
End With
With .Items
h = .AddItem("Root")
.FormatCell(h,1) = "'<none>'"
.CellValue(.InsertItem(h,,"Child 1"),1) = 10
.CellValue(.InsertItem(h,,"Child 2"),1) = 15
.CellValue(.InsertItem(h,,"Child 3"),1) = 25
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
652
|
I am using the FormatColumn to display the current currency, but would like hide some values. Is it possible
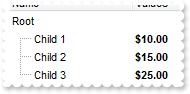
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.MarkSearchColumn = False
With .Columns
.Add "Name"
With .Add("Values")
.SortType = 1
.AllowSizing = False
.Width = 64
.FormatColumn = "((0:=dbl(value)) < 10? '<fgcolor=808080><font ;7>' :'<b>') + currency(=:0)"
.Def(17) = 1
End With
End With
With .Items
h = .AddItem("Root")
.FormatCell(h,1) = " "
.CellValue(.InsertItem(h,,"Child 1"),1) = 10
.CellValue(.InsertItem(h,,"Child 2"),1) = 15
.CellValue(.InsertItem(h,,"Child 3"),1) = 25
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
651
|
How can I specify an item to be always the first item
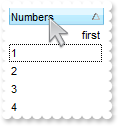
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.TreeColumnIndex = -1
.Columns.Add("Numbers").SortType = 1
With .Items
.AddItem 1
.AddItem 2
.AddItem 3
.AddItem 4
h = .AddItem("first")
.ItemPosition(h) = 0
.CellHAlignment(h,0) = 2
.SortableItem(h) = False
.SortChildren 0,0,False
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
650
|
How can I specify an item to be always the last item
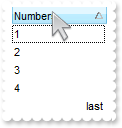
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.TreeColumnIndex = -1
.Columns.Add("Numbers").SortType = 1
With .Items
.AddItem 1
.AddItem 2
.AddItem 3
.AddItem 4
h = .AddItem("last")
.CellHAlignment(h,0) = 2
.SortableItem(h) = False
.SortChildren 0,0,True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
649
|
Can I allow sorting only the child items
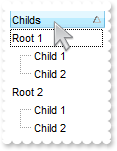
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add "Childs"
With .Items
h = .AddItem("Root 1")
.SortableItem(h) = False
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
.ExpandItem(h) = True
h = .AddItem("Root 2")
.SortableItem(h) = False
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
648
|
Can I specify a terminal item so it will mark the end of childs
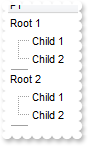
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ScrollBySingleLine = True
.Columns.Add "P1"
With .Items
h = .AddItem("Root 1")
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
.ExpandItem(h) = True
h = .InsertItem(h,"","")
.ItemDivider(h) = 0
.ItemDividerLineAlignment(h) = 1
.ItemHeight(h) = 2
.SelectableItem(h) = False
.SortableItem(h) = False
h = .AddItem("Root 2")
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
.ExpandItem(h) = True
h = .InsertItem(h,"","")
.ItemDivider(h) = 0
.ItemDividerLineAlignment(h) = 1
.ItemHeight(h) = 2
.SelectableItem(h) = False
.SortableItem(h) = False
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
647
|
Is it possible to specify an item being unsortable so its position won't be changed after sorting
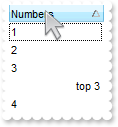
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.TreeColumnIndex = -1
.Columns.Add("Numbers").SortType = 1
With .Items
.AddItem 1
.AddItem 2
.AddItem 3
.AddItem 4
h = .AddItem("top 3")
.ItemPosition(h) = 3
.CellHAlignment(h,0) = 2
.SortableItem(h) = False
.SortChildren 0,0,False
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
646
|
Is it possible to move an item from a parent to another
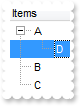
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
.Columns.Add "Items"
With .Items
.AddItem "A"
.AddItem "B"
.InsertItem .AddItem("C"),"","D"
.SetParent .FindItem("D",0),.FindItem("A",0)
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
645
|
How can I change the identation for an item

<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
.Columns.Add "Items"
With .Items
.AddItem "A"
.AddItem "B"
.InsertItem .AddItem("C"),"","D"
.SetParent .FindItem("D",0),0
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
644
|
How can I arrange the control's header on multiple levels
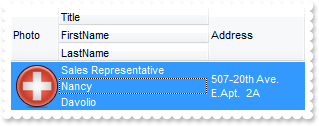
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.DefaultItemHeight = 48
With .Columns
.Add("Title").Visible = False
.Add("FirstName").Visible = False
.Add("LastName").Visible = False
.Add("Photo").Visible = False
With .Add("Address")
.Visible = False
.Def(16) = False
End With
With .Add("Personal Info")
.FormatLevel = "3:48,(0/1/2),4:96"
.Def(32) = "3:48,(0/1/2),4:96"
End With
End With
With .Items
h = .AddItem("Sales Representative")
.CellValue(h,1) = "Nancy"
.CellValue(h,2) = "Davolio"
.CellPicture(h,3) = Grid1.ExecuteTemplate("loadpicture(`c:\exontrol\images\zipdisk.gif`)")
.CellValue(h,4) = "507-20th Ave. E.Apt. 2A"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
643
|
How can I filter programatically using more columns
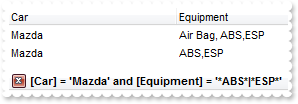
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
With .Columns
.Add "Car"
.Add "Equipment"
End With
With .Items
.CellValue(.AddItem("Mazda"),1) = "Air Bag"
.CellValue(.AddItem("Toyota"),1) = "Air Bag,Air condition"
.CellValue(.AddItem("Ford"),1) = "Air condition"
.CellValue(.AddItem("Nissan"),1) = "Air Bag,ABS,ESP"
.CellValue(.AddItem("Mazda"),1) = "Air Bag, ABS,ESP"
.CellValue(.AddItem("Mazda"),1) = "ABS,ESP"
End With
With .Columns.Item("Car")
.FilterType = 240
.Filter = "Mazda"
End With
With .Columns.Item("Equipment")
.FilterType = 3
.Filter = "*ABS*|*ESP*"
End With
.ApplyFilter
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
642
|
How can I show the ticks for a single slider field
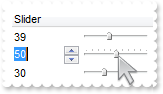
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add("Slider").Editor.EditType = 20
With .Items
.AddItem 10
With .CellEditor(.AddItem(20),0)
.EditType = 20
.Option(53) = 10
End With
.AddItem 30
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
641
|
Is it possible to show ticks for slider fields
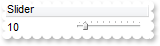
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
With .Columns.Add("Slider").Editor
.EditType = 20
.Option(53) = 10
End With
.Items.AddItem 10
End With
End Function
</SCRIPT>
</BODY>
|
640
|
Is it possible to colour a particular column, I mean the cell's foreground color
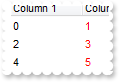
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
With .ConditionalFormats.Add("1")
.ForeColor = RGB(255,0,0)
.ApplyTo = 1 ' &H1
End With
.MarkSearchColumn = False
With .Columns
.Add "Column 1"
.Add "Column 2"
End With
With .Items
.CellValue(.AddItem(0),1) = 1
.CellValue(.AddItem(2),1) = 3
.CellValue(.AddItem(4),1) = 5
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
639
|
Is it possible to colour a particular column for specified values
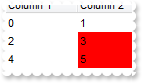
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
With .ConditionalFormats.Add("int(%1) in (3,4,5)")
.BackColor = RGB(255,0,0)
.ApplyTo = 1 ' &H1
End With
.MarkSearchColumn = False
With .Columns
.Add "Column 1"
.Add "Column 2"
End With
With .Items
.CellValue(.AddItem(0),1) = 1
.CellValue(.AddItem(2),1) = 3
.CellValue(.AddItem(4),1) = 5
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
638
|
Is it possible to colour a particular column
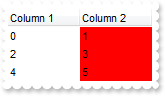
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.MarkSearchColumn = False
With .Columns
.Add "Column 1"
.Add("Column 2").Def(4) = 255
End With
With .Items
.CellValue(.AddItem(0),1) = 1
.CellValue(.AddItem(2),1) = 3
.CellValue(.AddItem(4),1) = 5
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
637
|
How do i get all the children items that are under a certain parent Item handle
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
.Columns.Add "P"
With .Items
h = .AddItem("Root")
.InsertItem h,,"Child 1"
.InsertItem h,,"Child 2"
.ExpandItem(h) = True
End With
With .Items
hChild = .ItemChild(.FirstVisibleItem)
alert( .CellValue(hChild,0) )
alert( .CellValue(.NextSiblingItem(hChild),0) )
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
636
|
Is is possible to use HTML tags to display in the filter caption
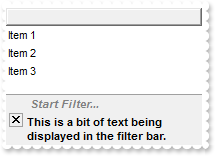
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.FilterBarPromptVisible = 1
.FilterBarCaption = "This is a bit of text being displayed in the filter bar."
.Columns.Add ""
With .Items
.AddItem "Item 1"
.AddItem "Item 2"
.AddItem "Item 3"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
635
|
How can I find the number of items after filtering
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.Columns.Add ""
With .Items
h = .AddItem("")
.CellValue(h,0) = .VisibleItemCount
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
634
|
How can I change the filter caption
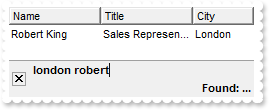
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptType = 12801 ' FilterPromptEnum.exFilterPromptWords Or FilterPromptEnum.exFilterPromptContainsAll
.FilterBarPromptPattern = "london robert"
.FilterBarCaption = "<r>Found: ... "
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
633
|
While using the filter prompt is it is possible to use wild characters
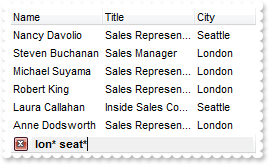
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptType = 16
.FilterBarPromptPattern = "lon* seat*"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
632
|
How can I list all items that contains any of specified words, not necessary at the beggining
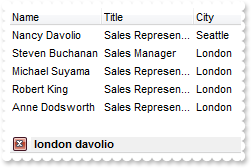
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptType = 4610 ' FilterPromptEnum.exFilterPromptStartWords Or FilterPromptEnum.exFilterPromptContainsAny
.FilterBarPromptPattern = "london davolio"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
631
|
How can I list all items that contains any of specified words, not strings
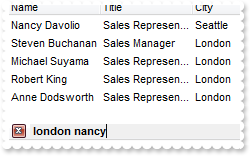
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptType = 12802 ' FilterPromptEnum.exFilterPromptWords Or FilterPromptEnum.exFilterPromptContainsAny
.FilterBarPromptPattern = "london nancy"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
630
|
How can I list all items that contains all specified words, not strings
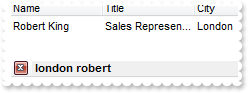
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptType = 12801 ' FilterPromptEnum.exFilterPromptWords Or FilterPromptEnum.exFilterPromptContainsAll
.FilterBarPromptPattern = "london robert"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
629
|
I've noticed that the filtering by prompt is not case sensitive, is is possible to make it case sensitive
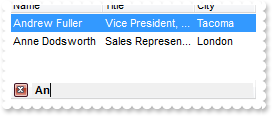
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptType = 258 ' FilterPromptEnum.exFilterPromptCaseSensitive Or FilterPromptEnum.exFilterPromptContainsAny
.FilterBarPromptPattern = "Anne"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
628
|
Is it possible to list only items that ends with any of specified strings
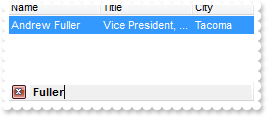
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptType = 4
.FilterBarPromptColumns = "0"
.FilterBarPromptPattern = "Fuller"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
627
|
Is it possible to list only items that ends with any of specified strings
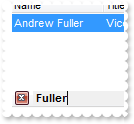
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptType = 4
.FilterBarPromptColumns = "0"
.FilterBarPromptPattern = "Fuller"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
626
|
Is it possible to list only items that starts with any of specified strings
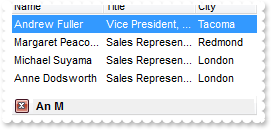
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptType = 3
.FilterBarPromptColumns = "0"
.FilterBarPromptPattern = "An M"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
625
|
Is it possible to list only items that starts with specified string
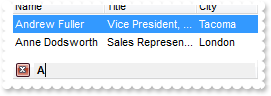
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptType = 3
.FilterBarPromptColumns = "0"
.FilterBarPromptPattern = "A"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
624
|
How can I specify that the list should include any of the seqeunces in the pattern
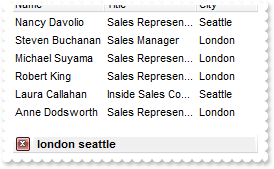
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptType = 2
.FilterBarPromptPattern = "london seattle"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
623
|
How can I specify that all sequences in the filter pattern must be included in the list
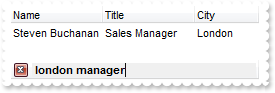
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptType = 1
.FilterBarPromptPattern = "london manager"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
622
|
How do I change at runtime the filter prompt
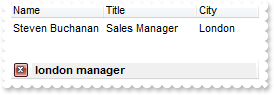
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptPattern = "london manager"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
621
|
How do I specify to filter only a single column when using the filter prompt
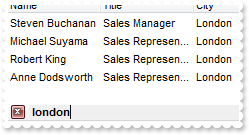
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPromptColumns = "2,3"
.FilterBarPromptPattern = "london"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
620
|
How do I change the prompt or the caption being displayed in the filter bar
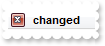
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
.FilterBarPrompt = "changed"
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
619
|
How do I enable the filter prompt feature
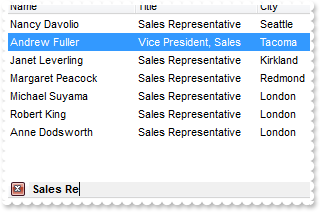
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.ColumnAutoResize = True
.ContinueColumnScroll = 0
.FocusColumnIndex = 1
.MarkSearchColumn = False
.SearchColumnIndex = 1
.FilterBarPromptVisible = 1
With .Columns
.Add("Name").Width = 96
.Add("Title").Width = 96
.Add "City"
End With
With .Items
h0 = .AddItem("Nancy Davolio")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Andrew Fuller")
.CellValue(h0,1) = "Vice President, Sales"
.CellValue(h0,2) = "Tacoma"
.SelectItem(h0) = True
h0 = .AddItem("Janet Leverling")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Kirkland"
h0 = .AddItem("Margaret Peacock")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "Redmond"
h0 = .AddItem("Steven Buchanan")
.CellValue(h0,1) = "Sales Manager"
.CellValue(h0,2) = "London"
h0 = .AddItem("Michael Suyama")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Robert King")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
h0 = .AddItem("Laura Callahan")
.CellValue(h0,1) = "Inside Sales Coordinator"
.CellValue(h0,2) = "Seattle"
h0 = .AddItem("Anne Dodsworth")
.CellValue(h0,1) = "Sales Representative"
.CellValue(h0,2) = "London"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
618
|
How can I control the colors that can be applied to an EBN part
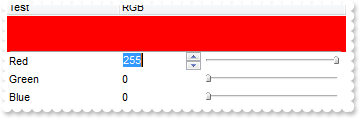
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_Change(Item,ColIndex,NewValue)
With Grid1
With .Items
.ItemBackColor(.FirstVisibleItem) = NewValue
End With
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
With .VisualAppearance
.Add 2,"c:\exontrol\images\normal.ebn"
.Add 1,"CP:2 10 3 -10 -5"
End With
.SelBackColor = .BackColor
.SelForeColor = .ForeColor
.ScrollBySingleLine = True
.TreeColumnIndex = -1
With .Columns
With .Add("Test")
.Width = 32
End With
With .Add("RGB")
With .Editor
.EditType = 20
.Option(44) = 255
.Option(41) = -60
End With
End With
End With
With .Items
h = .AddItem("")
.CellHAlignment(h,0) = 1
.ItemDivider(h) = 0
.ItemBackColor(h) = &H1000000
.ItemHeight(h) = 36
.SelectableItem(h) = False
h = .InsertItem(0,1,"Red")
.CellValue(h,1) = 255
h = .InsertItem(0,255,"Green")
.CellValue(h,1) = 255
h = .InsertItem(0,65536,"Blue")
.CellValue(h,1) = 255
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
617
|
I know this is fairly basic, but could you send me a sample that places a tree in the first column
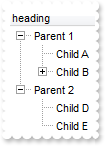
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
.Columns.Add "heading"
With .Items
h = .AddItem("Parent 1")
.InsertItem h,,"Child A"
.InsertItem .InsertItem(h,,"Child B"),,"GrandChild C"
.ExpandItem(h) = True
h = .AddItem("Parent 2")
.InsertItem h,,"Child D"
.InsertItem h,,"Child E"
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
616
|
How can I get the caption of focused item
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_SelectionChanged()
With Grid1
With .Items
alert( "Handle" )
alert( .FocusItem )
alert( "Caption" )
alert( .CellCaption(.FocusItem,0) )
End With
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
.Columns.Add "Items"
With .Items
h = .AddItem("R1")
.InsertItem h,,"Cell 1.1"
.InsertItem h,,"Cell 1.2"
.ExpandItem(h) = True
h = .AddItem("R2")
.InsertItem h,,"Cell 2.1"
.InsertItem h,,"Cell 2.2"
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
615
|
How can I get the caption of selected item
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_SelectionChanged()
With Grid1
With .Items
alert( "Handle" )
alert( .SelectedItem(0) )
alert( "Caption" )
alert( .CellCaption(.SelectedItem(0),0) )
End With
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
.Columns.Add "Items"
With .Items
h = .AddItem("R1")
.InsertItem h,,"Cell 1.1"
.InsertItem h,,"Cell 1.2"
.ExpandItem(h) = True
h = .AddItem("R2")
.InsertItem h,,"Cell 2.1"
.InsertItem h,,"Cell 2.2"
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
614
|
Is it possible to let users selects cells as in Excel
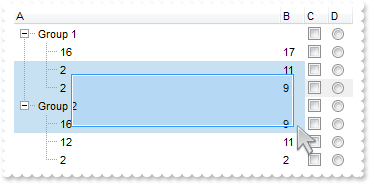
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.FullRowSelect = 1
.SingleSel = False
.ReadOnly = -1
.MarkSearchColumn = False
.ShowFocusRect = False
.LinesAtRoot = -1
.SelForeColor = RGB(0,0,0)
.SelBackColor = RGB(200,225,242)
With .Columns
.Add "A"
With .Add("B")
.AllowSizing = False
.Width = 24
End With
With .Add("C")
.AllowSizing = False
.Width = 24
.Def(0) = 1
.PartialCheck = True
End With
With .Add("D")
.AllowSizing = False
.Width = 24
.Def(1) = 1
End With
End With
With .Items
h = .InsertItem(,,"Group 1")
h1 = .InsertItem(h,,16)
.CellValue(h1,1) = 17
h1 = .InsertItem(h,,2)
.CellValue(h1,1) = 11
h1 = .InsertItem(h,,2)
.ItemBackColor(h1) = RGB(240,240,240)
.CellValue(h1,1) = 9
.ExpandItem(h) = True
h = .InsertItem(,,"Group 2")
.CellValueFormat(h,2) = 1
h1 = .InsertItem(h,,16)
.CellValue(h1,1) = 9
h1 = .InsertItem(h,,12)
.CellValue(h1,1) = 11
h1 = .InsertItem(h,,2)
.CellValue(h1,1) = 2
.ExpandItem(h) = True
.SelectItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
613
|
Is it possible to change the style for the vertical or horizontal grid lines, in the list area

<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.DrawGridLines = -1
.GridLineStyle = 33 ' GridLinesStyleEnum.exGridLinesVSolid Or GridLinesStyleEnum.exGridLinesHDot4
.Columns.Add "C1"
.Columns.Add "C2"
.Columns.Add "C3"
With .Items
h = .AddItem("Item 1")
.CellValue(h,1) = "SubItem 1.2"
.CellValue(h,2) = "SubItem 1.3"
h = .AddItem("Item 2")
.CellValue(h,1) = "SubItem 2.2"
.CellValue(h,2) = "SubItem 2.3"
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
612
|
Is it possible to change the style for the grid lines, for instance to be solid not dotted
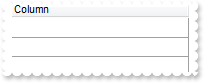
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.DrawGridLines = -1
.GridLineStyle = 48
.Columns.Add "Column"
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
611
|
I have some buttons added on the control's scroll bar, how can I can know when the button is being clicked
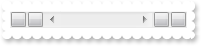
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_ScrollButtonClick(ScrollBar,ScrollPart)
With Grid1
alert( ScrollBar )
alert( ScrollPart )
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.ScrollPartVisible(1,32768) = True
.ScrollPartVisible(1,16384) = True
.ScrollPartVisible(1,1) = True
.ScrollPartVisible(1,2) = True
.ScrollBars = 5
End With
End Function
</SCRIPT>
</BODY>
|
610
|
How do I get notified once the user clicks a hyperlink created using the anchor HTML tag
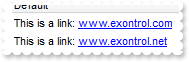
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_AnchorClick(AnchorID,Options)
With Grid1
alert( AnchorID )
alert( Options )
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.Columns.Add("Default").Def(17) = 1
With .Items
.AddItem "This is a link: <aex.com;1>www.exontrol.com</a>"
.AddItem "This is a link: <aex.net;2>www.exontrol.net</a>"
End With
End With
End Function
</SCRIPT>
</BODY>
|
609
|
Is it possible to start editing a cell when double click it
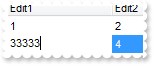
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_DblClick(Shift,X,Y)
With Grid1
.Edit
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.AutoEdit = False
.MarkSearchColumn = False
.Columns.Add("Edit1").Editor.EditType = 1
.Columns.Add("Edit2").Editor.EditType = 1
With .Items
.CellValue(.AddItem(1),1) = 2
End With
With .Items
.CellValue(.AddItem(3),1) = 4
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
608
|
Is it possible to disable standard single-click behavior for this column, so I manually could call Edit() when needed
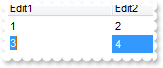
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_DblClick(Shift,X,Y)
With Grid1
.Edit
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.AutoEdit = False
.MarkSearchColumn = False
.Columns.Add("Edit1").Editor.EditType = 1
.Columns.Add("Edit2").Editor.EditType = 1
With .Items
.CellValue(.AddItem(1),1) = 2
End With
With .Items
.CellValue(.AddItem(3),1) = 4
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
607
|
How can I get or restore the old or previously value for the cell being changed
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_Change(Item,ColIndex,NewValue)
With Grid1
alert( "Old-Value:" )
alert( .Items.CellValue(Item,ColIndex) )
alert( "New-Value:" )
alert( NewValue )
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.MarkSearchColumn = False
.Columns.Add("Edit1").Editor.EditType = 1
.Columns.Add("Edit2").Editor.EditType = 1
With .Items
.CellValue(.AddItem(1),1) = 2
End With
With .Items
.CellValue(.AddItem(3),1) = 4
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
606
|
How can I get the item from the cursor
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_MouseMove(Button,Shift,X,Y)
With Grid1
h = .ItemFromPoint(-1,-1,c,hit)
alert( "Handle" )
alert( h )
alert( "Index" )
alert( .Items.ItemToIndex(h) )
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
.DrawGridLines = 1
.Columns.Add "Items"
With .Items
h = .AddItem("R1")
.InsertItem h,,"Cell 1.1"
.InsertItem h,,"Cell 1.2"
.ExpandItem(h) = True
h = .AddItem("R2")
.InsertItem h,,"Cell 2.1"
.InsertItem h,,"Cell 2.2"
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
605
|
How can I get the column from the cursor, not only in the header
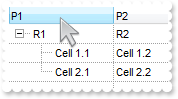
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_MouseMove(Button,Shift,X,Y)
With Grid1
alert( .ColumnFromPoint(-1,0) )
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
.Columns.Add "P1"
.Columns.Add "P2"
.DrawGridLines = -1
With .Items
h = .AddItem("R1")
.CellValue(h,1) = "R2"
.CellValue(.InsertItem(h,,"Cell 1.1"),1) = "Cell 1.2"
.CellValue(.InsertItem(h,,"Cell 2.1"),1) = "Cell 2.2"
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
604
|
How can I get the column from the cursor
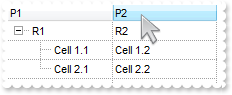
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_MouseMove(Button,Shift,X,Y)
With Grid1
alert( .ColumnFromPoint(-1,-1) )
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
.DrawGridLines = -1
.Columns.Add "P1"
.Columns.Add "P2"
With .Items
h = .AddItem("R1")
.CellValue(h,1) = "R2"
.CellValue(.InsertItem(h,,"Cell 1.1"),1) = "Cell 1.2"
.CellValue(.InsertItem(h,,"Cell 2.1"),1) = "Cell 2.2"
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
603
|
How can I get the cell's caption from the cursor
<BODY onload="Init()">
<SCRIPT LANGUAGE="VBScript">
Function Grid1_MouseMove(Button,Shift,X,Y)
With Grid1
h = .ItemFromPoint(-1,-1,c,hit)
alert( .Items.CellCaption(h,c) )
End With
End Function
</SCRIPT>
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.LinesAtRoot = -1
.Columns.Add "Items"
With .Items
h = .AddItem("R1")
.InsertItem h,,"Cell 1.1"
.InsertItem h,,"Cell 1.2"
.ExpandItem(h) = True
h = .AddItem("R2")
.InsertItem h,,"Cell 2.1"
.InsertItem h,,"Cell 2.2"
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
602
|
How can I customize the items based on the values in the cells
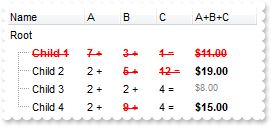
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.MarkSearchColumn = False
With .ConditionalFormats
With .Add("%1 >4")
.Bold = True
.StrikeOut = True
.ForeColor = RGB(255,0,0)
.ApplyTo = -1
End With
With .Add("%2 > 4")
.Bold = True
.StrikeOut = True
.ForeColor = RGB(255,0,0)
.ApplyTo = 2 ' &H2
End With
With .Add("%3 > 4")
.Bold = True
.StrikeOut = True
.ForeColor = RGB(255,0,0)
.ApplyTo = 3 ' &H3
End With
End With
With .Columns
.Add "Name"
With .Add("A")
.SortType = 1
.AllowSizing = False
.Width = 36
.FormatColumn = "len(value) ? value + ' +'"
.Editor.EditType = 4
End With
With .Add("B")
.SortType = 1
.AllowSizing = False
.Width = 36
.FormatColumn = "len(value) ? value + ' +'"
.Editor.EditType = 4
End With
With .Add("C")
.SortType = 1
.AllowSizing = False
.Width = 36
.FormatColumn = "len(value) ? value + ' ='"
.Editor.EditType = 4
End With
With .Add("A+B+C")
.SortType = 1
.AllowSizing = False
.Width = 64
.ComputedField = "%1+%2+%3"
.FormatColumn = "((0:=dbl(value)) < 10? '<fgcolor=808080><font ;7>' :'<b>') + currency(=:0)"
.Def(17) = 1
End With
End With
With .Items
h = .AddItem("Root")
.CellValueFormat(h,4) = 2
h1 = .InsertItem(h,,"Child 1")
.CellValue(h1,1) = 7
.CellValue(h1,2) = 3
.CellValue(h1,3) = 1
h1 = .InsertItem(h,,"Child 2")
.CellValue(h1,1) = 2
.CellValue(h1,2) = 5
.CellValue(h1,3) = 12
h1 = .InsertItem(h,,"Child 3")
.CellValue(h1,1) = 2
.CellValue(h1,2) = 2
.CellValue(h1,3) = 4
h1 = .InsertItem(h,,"Child 4")
.CellValue(h1,1) = 2
.CellValue(h1,2) = 9
.CellValue(h1,3) = 4
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|
601
|
Is it is possible to have a column computing values from other columns
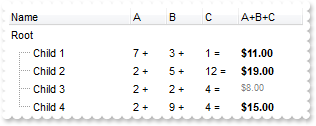
<BODY onload="Init()">
<OBJECT CLASSID="clsid:101EE60F-7B07-48B0-A13A-F32BAE7DA165" id="Grid1"></OBJECT>
<SCRIPT LANGUAGE="VBScript">
Function Init()
With Grid1
.BeginUpdate
.MarkSearchColumn = False
With .Columns
.Add "Name"
With .Add("A")
.SortType = 1
.AllowSizing = False
.Width = 36
.FormatColumn = "len(value) ? value + ' +'"
.Editor.EditType = 4
End With
With .Add("B")
.SortType = 1
.AllowSizing = False
.Width = 36
.FormatColumn = "len(value) ? value + ' +'"
.Editor.EditType = 4
End With
With .Add("C")
.SortType = 1
.AllowSizing = False
.Width = 36
.FormatColumn = "len(value) ? value + ' ='"
.Editor.EditType = 4
End With
With .Add("A+B+C")
.SortType = 1
.AllowSizing = False
.Width = 64
.ComputedField = "%1+%2+%3"
.FormatColumn = "((0:=dbl(value)) < 10? '<fgcolor=808080><font ;7>' :'<b>') + currency(=:0)"
.Def(17) = 1
End With
End With
With .Items
h = .AddItem("Root")
.CellValueFormat(h,4) = 2
h1 = .InsertItem(h,,"Child 1")
.CellValue(h1,1) = 7
.CellValue(h1,2) = 3
.CellValue(h1,3) = 1
h1 = .InsertItem(h,,"Child 2")
.CellValue(h1,1) = 2
.CellValue(h1,2) = 5
.CellValue(h1,3) = 12
h1 = .InsertItem(h,,"Child 3")
.CellValue(h1,1) = 2
.CellValue(h1,2) = 2
.CellValue(h1,3) = 4
h1 = .InsertItem(h,,"Child 4")
.CellValue(h1,1) = 2
.CellValue(h1,2) = 9
.CellValue(h1,3) = 4
.ExpandItem(h) = True
End With
.EndUpdate
End With
End Function
</SCRIPT>
</BODY>
|